Learn how to receive SMS with PHP using the code samples found on this page.
Receiving SMS using PHP is helpful to send status updates, trigger commands, or advanced communication integration. This page will help you to get started and offers a script for receiving sms.
Receive SMS using PHP POST
PHP will handle all of the SMS it receives using POST. Each SMS will have at least three parameters attached to it with data that your server can access. Take a look at the parameters and their contents below.
'to' => '15554443332', 'from' => '15554443333', 'msg' => 'Received Message Here', 'attach' => 'URL to Optional MMS attachment'
- to contains a value that will match an active number within your SMS Arc account.
- from parameter is used to hold the phone number which sent the message to PHP.
- msg is the content of the SMS. PHP will receive these in 160 character segments.
- attach willl contain a comma separated URL for each attachment (optional)
PHP Script for Receiving SMS
Take a look at the following sample code. You can copy and paste the script for receiving SMS using PHP and begin working with it in seconds. There are no dependencies required, or additional software needed: Our PHP script for receiving SMS is compatible with all web servers and PHP versions.
<?php ////// //Example A: send SMS data to Email ////// $to = 'YOUR_EMAIL@HERE.com'; $subject = 'Received SMS on '.date("F j, Y, g:i a"); //prepare the message $message = 'New SMS received on '.date("F j, Y, g:i a").' <br>To: '.$_POST['to'].' <br>From: '.$_POST['from'].' <br>Message: '.$_POST['message']; //send the mail mail($to, $subject, $message); ////// //Example B: save SMS data to log file ////// $fp = fopen('sms.log', 'a'); $sms_data = print_r($_POST, TRUE); fwrite($fp, $sms_data); fclose($fp); ?>
When an SMS is received by PHP, you can handle the data and do anything you want with it: store the message in your SQL database, send an email, or perform custom functions. In order to get started, you need to let SMS Arc know your endpoint URL when managing your phone numbers.
Selecting a PHP Endpoint for Receiving SMS
In order to begin receiving SMS with PHP using the script provided above, you need to configure your number with SMS Arc. We provide a simple to use platform that will let you get started in seconds. The first step to receiving text messages with PHP is to activate a number with SMS Arc.
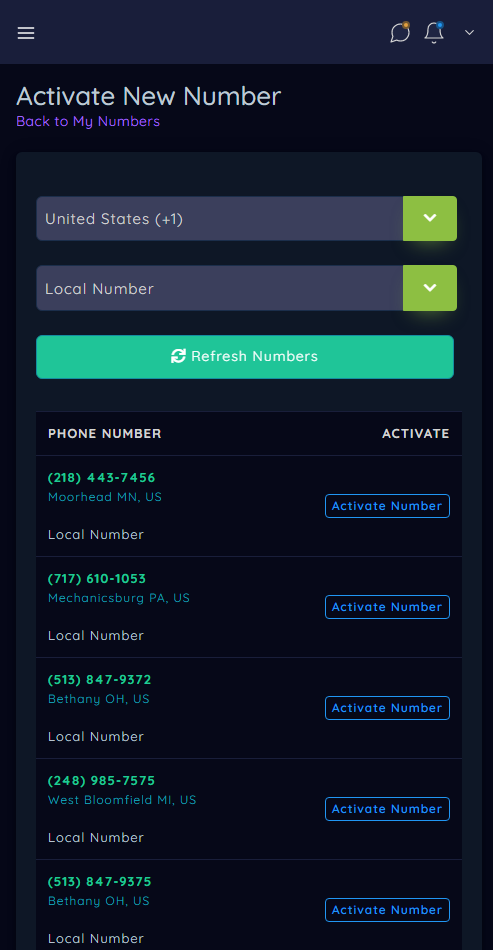

Note that Toll-Free numbers can only receive SMS and do not support MMS messages. Use a local number if you need to receive MMS with PHP.
Configure Your Phone Number to Forward to PHP
Once you have activated your phone number with SMS Arc, you can use the interface to configure the URL Endpoint used for receiving SMS.
This is the URL that SMS Arc will use to POST all SMS data to. You can use any URL on your webserver, and can even handle the SMS with languages in addition to PHP.
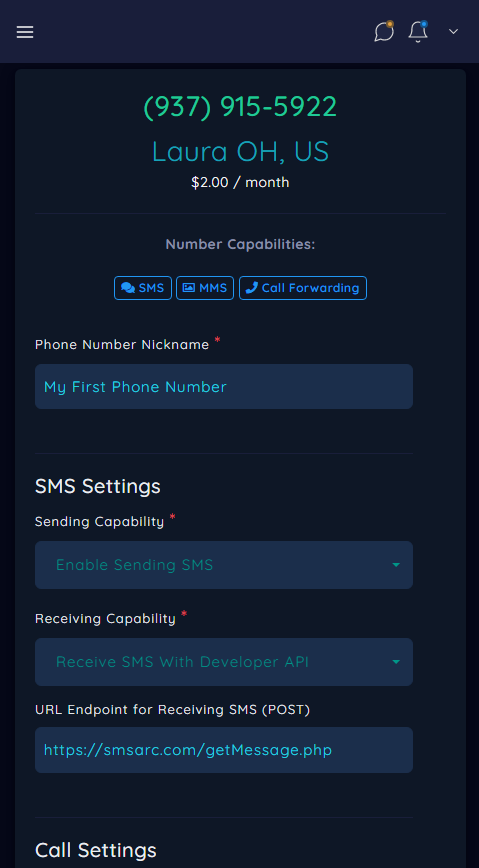
Once you specify the URL Endpoint for sending SMS data, PHP will begin receiving all SMS that received by the number you have configured. After setting up the PHP script for receiving SMS you can send a text message to your number to test it out.
What Number does PHP use for Receiving SMS?
You can use multiple numbers for receiving SMS with PHP, and any number that you activate within the SMS Arc platform can be used for receiving SMS with PHP or other programming languages.
Need more than one number?
SMS Arc allows you to activate as many local or toll-free numbers as your platform needs with one click. You can setup a number for each purpose within your project or business.
Not sure where to start?
How To Receive SMS with PHP
We are ready to help if you have you have questions about setting up our PHP script or other questions related to receiving SMS with PHP. Contact support or take a look at our frequently asked questions and answers that we have provided below.
Do I need to install anything on my web server?
You do not need any software or dependencies to receive SMS with PHP.
SMS Arc allows you to handle receiving SMS as easily as any web form.
Our platform will handle all of the heavy lifting, and send the SMS data to you.
How does PHP receive the SMS?
PHP uses POST to recieve the SMS data that we send after receiving a text message on one of your active numbers.
How Fast can PHP receive SMS?
All messages are sent to PHP instantly, with the same speed as regular text messaging. You can test it out yourself!
What is the longest text message PHP can receive?
Like with regular texting, PHP can receive SMS messages up to 160 characters long. Any messages longer than 160 characters may be broken up depending on the carrier of the sending phone number.
Can I receive MMS with PHP?
You can receive MMS with PHP using the script provide above. Any MMS attachments will appear as a value within the attach parameter sent to PHP. PHP will receive MMS as comma separated URLS, one for each attachment.